7 smart ways to use AI in WordPress development
Artificial Intelligence (AI) tools like ChatGPT, Claude, and Cursor are quietly becoming part of the daily toolkit for WordPress developers. Whether you’re building custom plugins, working with Gutenberg blocks, or automating tasks with WP-CLI, AI can help you write, debug, and refactor code faster without sacrificing quality.
This guide walks you through seven practical ways developers use AI to streamline real WordPress workflows.
Let’s get into them.
1. Writing and debugging custom plugin code
One of the most obvious (and powerful) ways to use AI as a WordPress developer is to write and troubleshoot custom plugin code.
Whether you’re building something from scratch or dealing with a client’s legacy plugin that’s throwing fatal errors, tools like ChatGPT and Claude can seriously speed up your workflow.
Building a plugin boilerplate from scratch
You can use AI to scaffold the entire structure of a plugin, including the header, hooks, and file organization. Instead of reaching for that one old plugin you always copy-paste from, just describe what you want.
Here is an example prompt:
Create a WordPress plugin that registers a custom post type called "Event. "It should support title, editor, and thumbnail and have custom meta fields for date and location. Include code to register these meta fields using the REST API.
Claude does not just dump raw code. It gives:
- A full plugin scaffold, object-oriented and nicely structured.
- Inline comments throughout the code explaining each part.
- Proper indentation and spacing (you’d think that’s a given, but it’s not with all tools).
- REST-ready meta fields registered via
register_post_meta()
. - An admin UI with a meta box to capture the event date and location.
- And lots more.
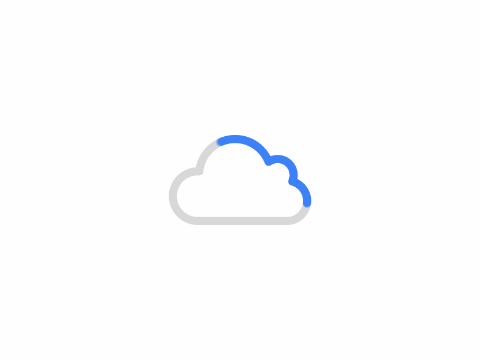
Debugging plugin errors
If you’re staring at a white screen or a fatal error from someone else’s plugin, AI can help you identify the problem quickly. ChatGPT (especially GPT-4) is good at explaining stack traces and spotting missing function calls, typos, or deprecated functions.
Here is an example prompt given to ChatGPT:
Here's an error I'm getting in a custom plugin:
"Uncaught Error: Call to undefined function get_field() in /wp-content/plugins/my-plugin/plugin.php on line 42"
What's wrong and how can I fix it?
And ChatGPT nailed it:
- It correctly identified that
get_field()
is an Advanced Custom Fields (ACF) function. - It listed all the common reasons for this error.
- It even suggested best practices like wrapping the function in a hook, like
init
orwp
, and checkingfunction_exists()
before calling it.
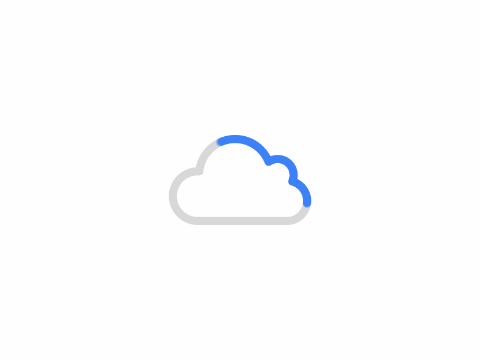
You can even paste entire plugin files into tools like Cursor and ask it to “audit the code for WordPress best practices,” or “rewrite this to follow modern PHP and WP coding standards.”
Modifying existing plugin functionality
Let’s say you’re handed a plugin that does 80% of what you need, but that last 20% matters. Maybe you need to tweak some output, hook into a form submission, or make it multisite-compatible.
Instead of digging through the codebase manually, you can use AI tools like Cursor or GitHub Copilot right inside your editor to make changes faster and safer. For example, this kind of prompt could help:
This plugin creates a custom post type for “Testimonials” and displays them using a shortcode. Modify it to also output the testimonial author’s name in bold below the content. Here’s the shortcode output function:
[...paste function...]
Or something like:
Update this plugin so that it doesn’t run on multisite installations. If it is a multisite, show an admin notice and deactivate the plugin.
AI will then:
- Locate the exact function or hook in the file (even if you’re not sure).
- Suggests the smallest change needed, rather than rewriting the whole thing.
- Keep the logic scoped to the plugin’s structure (especially if you’re using Cursor and it’s reading the entire codebase).
- If needed, it’ll add safety checks, like
is_multisite()
orfunction_exists()
.
It might even ask, “Do you want the author name to be optional? Should it come from post meta or a shortcode attribute?” — a good sign that it’s “thinking” in developer terms.
2. Creating custom Gutenberg blocks
Gutenberg block development can be a pain, especially if you’re not deep into React. It’s easy to get bogged down between the Webpack setup, block registration, and rendering logic. This is where AI tools can cut friction from the process.
Generate a custom block from scratch
I asked Claude to create a custom Gutenberg block called Testimonial Block, with support for a quote, author name, and author image:
Create a Gutenberg block called "Testimonial Block". It should have fields for a quote, author name, and author image. Show a preview in the editor and render it on the frontend using PHP. Output the block with basic markup and class names so I can style it later.
Claude nailed the structure. Instead of dumping everything into a single blob, it split the plugin into clear parts:
- PHP plugin file (
testimonial-block.php
) — registers the block usingregister_block_type()
. - JS file (
block.js
) — sets up the block UI usingTextControl
,MediaUpload
,useBlockProps
, etc. - CSS files (
editor.css
andstyle.css
) — styles scoped for both the editor and the frontend
It also walked through where to save each file and how to structure the folder inside /wp-content/plugins/
, making it easy to test immediately.
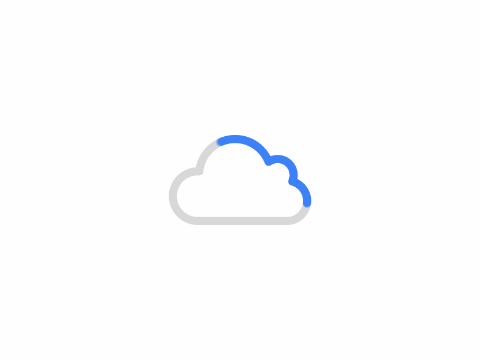
If you’re working with native blocks and don’t feel like setting up @wordpress/scripts
from scratch every time, this kind of AI assist gets you 80% of the way there. You can always customize the markup or field structure later.
If you want to change the layout, you can just tell Claude: “Make the author image appear above the quote instead of beside it,” or “Replace the MediaUpload with an external image URL input instead.”
Modifying existing blocks
Like when generating a block from scratch, you can also use Claude or ChatGPT to tweak existing Gutenberg blocks, which is especially useful when you’re working on a project someone else started or revisiting a block you wrote months ago.
For example, let’s say you have a block with a simple text input and want to add a toggle to control whether the output should be highlighted or not. Instead of manually digging through edit()
and save()
or the PHP render_callback
, you can just give AI the relevant part of the block and ask:
Here’s the edit() function for my Gutenberg block. Add a ToggleControl labeled "Highlight" that adds a CSS class "highlighted" to the block wrapper if it's turned on:
[...paste function...]
It’s also smart enough to follow your existing code style. So, if your block uses useBlockProps()
, it keeps that; if it’s rendering raw div
markup, it rolls with that instead of trying to rewrite your layout.
3. Creating WP-CLI commands for automation
As a WordPress developer, WP-CLI is one of those “level up” tools. It lets you script WordPress like a proper app instead of clicking around the admin panel or writing temporary admin pages just to perform a bulk action.
AI removes all the overhead, so you no longer have to dig through WP-CLI docs, guess the class format, copy old code from another project, and tweak it for 30 minutes.
Let’s say you want to publish all posts in bulk with a specific meta key. You can use the following prompt:
Write a custom WP-CLI command called `publish_scheduled_events` that loops through all posts of type "event" where the custom meta key "event_date" is in the past and publishes them.
AI will give you back a class with WP_CLI::add_command()
registered properly alongside a method that uses WP_Query
with a meta_query
filter, and lots more. Most times, the code is production-ready, minus the actual meta key value comparison tweak, which you can ask for in a follow-up.
You can also ask for WP-CLI commands to handle tasks like:
- Clearing transients
- Re-saving permalinks
- Regenerating image sizes
- Syncing options across environments
- Running custom import jobs on schedule
For example, see the following prompt:
Write a WP-CLI command that deletes all expired transients in the wp_options table and logs how many were deleted.
Also, if you’re already writing WP-CLI commands but something’s off (maybe it does not recognize arguments or you’re getting weird output), just paste the code and ask:
This WP-CLI command isn’t parsing the --user_id argument correctly. What’s wrong?
4. Optimizing SQL queries in WP queries or custom database code
WordPress developers often deal with queries that look fine until they start running on a real site with thousands of posts and a bloated wp_postmeta
table. That’s when performance drops, and things get ugly fast.
The good news is that tools like ChatGPT, Claude, and even Cursor (when working in a full codebase) can review your SQL or WP_Query
config and point out inefficient patterns, or help you refactor queries entirely.
Spot bottlenecks in WP_Query config
Let’s say you’ve written a complex WP_Query
to display upcoming events with custom meta fields, and it’s loading slowly. You can ask:
Here’s a WP_Query for events sorted by a custom meta field "event_date". It’s slow when there are lots of events. How can I optimize it?
[...paste the WP_Query args...]
And AI might respond with:
- A reminder that
meta_query
is not indexed, so querying large datasets will always be expensive. - A suggestion to avoid using
orderby => 'meta_value'
if possible. - Advice to store a normalized date in a custom DB column or a taxonomy for better performance.
- It may even suggest rewriting the logic to use
pre_query
hooks to alter SQL directly.
Analyze and refactor raw SQL
Sometimes you’re bypassing WP_Query
altogether — maybe for reporting, analytics, or plugin logic. You’ve written a raw SELECT
query that joins wp_posts
and wp_postmeta
, but it’s slow or returning duplicate results.
You can use a prompt like:
This SQL query is slow. Can you help me optimize it?
SELECT p.ID, p.post_title, m.meta_value
FROM wp_posts p
JOIN wp_postmeta m ON p.ID = m.post_id
WHERE m.meta_key = 'event_date'
AND m.meta_value >= CURDATE()
AND p.post_type = 'event'
AND p.post_status = 'publish'
Explain what a query is actually doing
If you’re handed some ancient plugin or theme code that runs a big SQL query (and no one knows what it does), you can drop it into ChatGPT or Claude and ask:
Explain what this WordPress SQL query is doing and tell me if it could be made more efficient:
[...query...]
AI will walk you through:
- What tables are being joined and why.
- What each
WHERE
clause is filtering. - Whether any part of the query is redundant.
- If the
LIMIT
,ORDER BY
, orGROUP BY
is a problem.
It’ll even explain bad things like SELECT *
, Cartesian joins, or inefficient regex in LIKE
clauses.
5. Generating unit/integration tests (PHPUnit) for plugins
Writing tests for WordPress code isn’t always straightforward. Bootstrapping the WP testing environment, mocking core functions, and figuring out what needs testing can be a lot.
AI tools are good at writing test cases, especially if you give them a function or class and ask for specific behavior to be tested.
Let’s say you’ve written a function that creates a custom post and saves some associated metadata. You want to test that it:
- Creates the post successfully.
- Assigns the correct post type.
- Saves meta fields correctly.
The following prompt can work:
Write PHPUnit tests for this function. It creates a custom post type "Event" and saves meta fields "event_date" and "event_location":
[...paste function...]
If you’ve got a plugin that saves settings via admin-post.php
, you can test that, too. Just feed the form handler function to AI and ask:
Write PHPUnit tests for this function that handles plugin settings form submissions. It saves an option based on POST data and checks a nonce.
If your plugin registers custom REST API routes, manually testing those is slow and error-prone. AI tools can also help you build tests that use wp_remote_get()
or rest_do_request()
directly:
Write a PHPUnit test that sends a GET request to my custom REST route `/wp-json/my-plugin/v1/data` and checks for a 200 response and valid JSON output.
Even basic tests catch issues early. When AI handles the boilerplate, you can focus on testing logic, not fiddling with setup. You don’t have to become a TDD purist — just ask: “What should I test in this function?” … and you’ll get ideas you probably overlooked. It makes testing less of a chore and more like an extension of development.
6. Refactoring or translating old code
If you’ve been working with WordPress for more than a few years, you’ve probably touched some jQuery-heavy code — inline scripts, global variables everywhere, weird timing issues, maybe even $(document).ready()
buried in PHP files.
The problem is that WordPress has moved on. Gutenberg uses React, themes are going block-based, and even admin UIs benefit from modern JS. Refactoring that jQuery into clean, modular JavaScript (or even React where it makes sense) can be painful — unless you use AI to speed it up.
Let’s say you have some old-school code like this:
jQuery(document).ready(function($) {
$('#open-modal').on('click', function() {
$('#my-modal').fadeIn();
});
$('.close-modal').on('click', function() {
$('#my-modal').fadeOut();
});
});
And you want to convert that into modern, dependency-free JS. Just ask:
Convert this jQuery code to modern vanilla JavaScript using addEventListener and class toggling instead of fadeIn/fadeOut:
[...paste code...]
Claude or ChatGPT will return:
document.addEventListener('DOMContentLoaded', function() {
document.getElementById('open-modal').addEventListener('click', function() {
document.getElementById('my-modal').classList.add('visible');
});
document.querySelectorAll('.close-modal').forEach(function(btn) {
btn.addEventListener('click', function() {
document.getElementById('my-modal').classList.remove('visible');
});
});
});
It’ll usually recommend adding styles like:
#my-modal {
display: none;
}
#my-modal.visible {
display: block;
}
This makes the code easier to maintain, loads faster, and doesn’t require jQuery on the frontend.
Also, let’s say you’re building or updating a Gutenberg block, and your old JS uses jQuery to inject markup dynamically. You want that logic moved into React so it can live inside edit()
properly.
The following prompt would work:
Translate this jQuery code that appends a div with post data into a React component for a Gutenberg block:
[...paste jQuery code...]
If your code uses WordPress APIs like wp.apiFetch
, AI knows how to integrate that as well — often suggesting better async patterns or handling errors more gracefully than legacy code.
Finally, let’s say you’re dealing with a plugin that has 300 lines of procedural JavaScript dumped into one <script>
tag. AI can help split it into logical parts using a prompt like:
Break this JavaScript into reusable functions and separate concerns. Put DOM setup, event handlers, and data logic into their own functions:
[...paste code...]
7. Making hosting and DevOps easier
WordPress development doesn’t stop at writing code — it includes everything from deployment to updates, performance, and hosting configuration. If you’re managing your sites on a platform like Kinsta, AI tools can help you move faster and make fewer mistakes in that ops layer, too.
For example, if you got a cryptic error from Kinsta’s PHP error logs or APM tool, you can paste it into ChatGPT and ask:
This error came from Kinsta’s PHP logs. Can you explain what it means and how to fix it?
It’ll help decode fatal errors, memory issues, or plugin conflicts faster than combing through docs or Stack Overflow.
If a piece of the Kinsta docs, a plugin README, or some .htaccess
rule doesn’t make sense, just drop it into Claude and say:
Explain this part to me like I’m a developer but unfamiliar with server config.
AI tools can also help you generate or review Git-based CI/CD workflows like GitHub Actions, GitLab CI, or Bitbucket Pipelines that deploy themes, sync files, or run database migrations via SSH on Kinsta. Just prompt:
Write a GitHub Actions workflow that deploys my WordPress theme to a Kinsta server over SSH after pushing to the main branch.
In short, AI becomes a layer between you and the time-consuming or unclear parts of hosting or DevOps — whether that’s reading logs, scripting deployments, or understanding documentation.
That said, handling hosting issues such as performance problems, errors, and server config still requires real expertise. If something breaks, it can be frustrating, time-sensitive, and costly for your business. That’s why Kinsta backs its platform with 24/7/365 support in 10 languages from expert engineers ready to help you troubleshoot, explain, and resolve WordPress server issues in a friendly, human way.
Summary
AI isn’t here to replace WordPress developers — it’s here to make us faster, our code cleaner, and less prone to boring mistakes.
The key is to treat AI like a junior dev — not a magic wand. Don’t expect it to nail everything in one giant prompt. Break the work into steps, review what it gives you, and build layer by layer. That’s how you stay in control while getting all the speed benefits AI has to offer.
Whether you’re writing custom plugins, optimizing performance, or deploying sites at scale, Kinsta provides the speed, tools, and expert support you need.
The post 7 smart ways to use AI in WordPress development appeared first on Kinsta®.
版权声明:
作者:zhangchen
链接:https://www.techfm.club/p/212581.html
来源:TechFM
文章版权归作者所有,未经允许请勿转载。
共有 0 条评论