How To Create a Headless WordPress Site With React.js
WordPress is one of the most popular content management systems (CMS), used by 810 million — that’s 41% of the whole internet! It’s the go-to choice for anyone who wants to quickly build a website because it’s simple, user-friendly, offers a wide variety of customization options, and also has a strong ecosystem of plugins and other resources.
One way to make the most out of WordPress is by going headless.
A headless CMS, also known as a headless system, is a type of backend CMS that’s solely used for managing content. This helps you integrate content into any system or website by just calling the APIs of the headless CMS.
However, this leaves the frontend to manage separately. This is where an API comes in.
APIs allow two or more different applications to exchange data. In this case, the API is being used to transfer the data from the CMS to a frontend website, providing more flexibility and faster performance.
In this article, we’ll explore what a headless CMS is, why you might want to build one, and how to set one up for WordPress.
What Is Headless WordPress?
A headless WordPress website is a type of site that mainly uses WordPress as a CMS, or content management system, and uses other frontend technologies such as React or Vue for the frontend.
The JavaScript libraries and frameworks are used to display the website contents. Therefore, a headless WordPress has a separate frontend and backend, and an API is used for communication.
In simpler terms, a headless architecture means that the CMS is used only for storing and managing your content, and it doesn’t care about the frontend of the website.
The frontend’s main job, on the other hand, is to display the content, and it in turn doesn’t care where the content is stored or managed, as long as it can reach it.
A headless WordPress has better performance since the frontend requests are handled by faster technologies such as React, and only the backend is managed by WordPress. The separation of frontend and backend makes it possible to scale components independently.
Pros and Cons of Headless WordPress
As with all development options, there are both benefits and drawbacks to going with a headless WordPress solution. It’s important to understand both before making a decision.
Pros of Headless WordPress
Some of the key benefits of a headless WordPress implementation include the following:
- Flexibility: Headless WordPress allows developers to create custom frontend experiences without being restricted by the traditional WordPress theme system. This means you can create unique user interfaces and experiences for specific needs.
- Performance: Separating the frontend from the backend of a WordPress site can make your website load faster and increase its performance, as the server is only delivering data via an API, rather than also rendering HTML for each request.
- Security: By separating the frontend and backend, headless WordPress can provide an extra layer of security by limiting access to the underlying WordPress codebase and database.
Cons of Headless WordPress
The drawbacks of headless WordPress can include:
- Lack of themes: Since headless WordPress does not rely on pre-built themes, you will need to create your own custom themes. This can be time-consuming and may require additional resources.
- Cost: Developing a headless WordPress site can be more expensive than a traditional WordPress site, as it requires more technical expertise and resources to set up and maintain.
- Plugin limitations: Some WordPress plugins may not work with headless WordPress, as they rely on WordPress themes to function properly.
What Is the WordPress REST API?
The WordPress REST API is used as an interface between the backend and frontend. With the API, data can be sent or retrieved from the site with ease, as the API has control access to the site’s data. It also ensures that only authorized users can interact with it.
The API can support a wide range of data formats, including JSON, which makes it easy to interact with the system.
WordPress REST API is a powerful tool for developers to create, update, or delete data in addition to creating custom functionality for sites or integrating with another service. Moreover, there are plugins available that extend the functionality of the API, such as integrating additional authentication methods.
What Is React?
React is a JavaScript library for building user interfaces. It is an open-source, reusable component-based frontend library that allows developers to build user interface (UI) components using declarative syntax.
React creates an interactive UI and renders the components when the data changes. The library is highly popular among developers looking to create complex, reusable components; manage state efficiently; and easily update the user interface in real time.
React’s strong developer community has created a set of tools, libraries, and resources to enhance the library’s functionality. Many organizations and businesses are adopting React as their technology for building complex, dynamic, and fast-performing web applications.
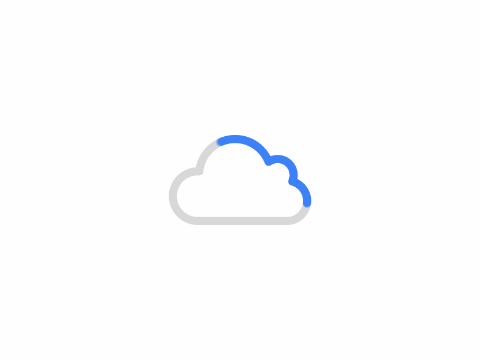
Why Use React?
React offers many benefits that make it an excellent choice for developing complex and dynamic web applications.
Here are some of the key advantages of using React:
- Declarative syntax: React uses a declarative approach to building UI components, making it easy to create reusable and highly efficient components.
- Large community and ecosystem: React has a large and active community of developers, which has led to the creation of a wide range of tools and libraries to enhance its functionality.
- Virtual DOM: React uses virtual DOM to update the UI in real-time. This means that when the state changes, It only updates the components that need to be changed, rather than re-rendering the entire page.
- Reusability: React.js offers reusable components that can be used across different applications, which significantly reduces development time and effort.
How To Create a Headless WordPress Site With React
Now it’s time to get our hands dirty and learn how to create and deploy a headless WordPress site with React.
Keep reading to dive in.
Prerequisites
Before you start with this tutorial, make sure you have:
- A good understanding of React
- Node.js v14 or higher installed on your machine
Step 1. Setting Up a WordPress Website
Let’s start by setting up the WordPress website, as this will serve as the data source for the React application. If you already have a WordPress website setup, you can skip this section; otherwise, just follow along.
In this tutorial, we will be using DevKinsta, a widely used fast, and reliable local development environment for creating, developing, and deploying WordPress sites. It’s completely free to use — simply download it from the official website and install it on your system.
Once the installation is completed, open the DevKinsta dashboard and click New WordPress site to create a new WordPress site.
Fill in the required inputs such and press the Create site button to continue.
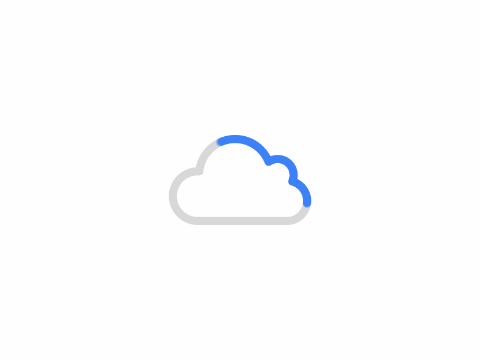
This could take a few minutes, but once your website is created, you can access it and its admin panel by clicking Open site” or WP Admin options respectively.
Next, to enable the JSON API, you’ll need to update the permalinks of your website.
In the WordPress admin dashboard, click on Settings, followed by Permalinks. Choose the Post name option and click Save Changes.
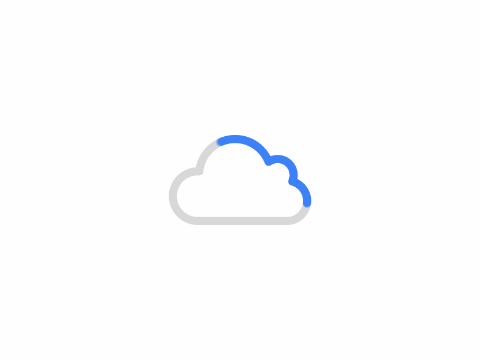
You can also use tools such as Postman to easily test and send requests to WordPress REST APIs.
Step 2: Setting Up a React Application
Now that we have our WordPress website set up, we can start working on the frontend. As mentioned above, in this tutorial we will be using React for the frontend of our application.
To get started, run the below code in your terminal to create a React application.
npm create vite@latest my-blog-app
cd my-blog-app
npm install
The above commands will create a React application and install the required dependencies.
We also need to install Axios, a JavaScript library for making HTTP requests. Run the below command to install it.
npm install axios
Now, in order to start the development server, you can run npm run dev
in the terminal. The server should then initialize your app at http://127.0.0.1:5173.
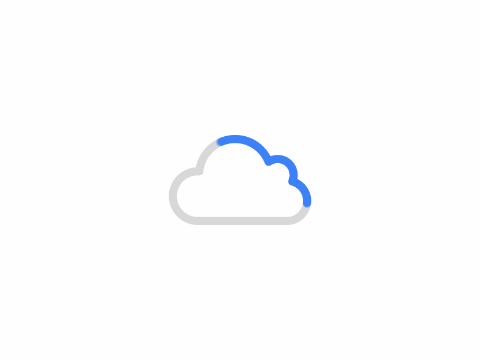
Next, open your project in your favorite code editor and delete any unnecessary files that you don’t need, such as the assets folder, index.css, and app.css.
You can also replace the code inside main.jsx and App.jsx with the following code:
// main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
// App.jsx
import React from 'react'
export default function App() {
return (
<App />
)
}
Step 3: Fetching Posts From WordPress
Now it’s time to fetch the posts from our WordPress website.
Inside the App.jsx, add the below state and import <code>useState</code> from React:
const [posts, setPosts] = useState([])
useState
is a built-in hook in React that is used to add states to a component, a state referring to data or a property.
posts
is used to get the data from the state, and setPosts
is used to add new data to post. We have also passed an empty array to state by default.
Next, add the following code after the state to fetch the posts from the WordPress REST API:
const fetchPosts = () => {
// Using axios to fetch the posts
axios
.get("http://headless-wordpress-website.local/wp-json/wp/v2/posts")
.then((res) => {
// Saving the data to state
setPosts(res.data);
});
}
// Calling the function on page load
useEffect(() => {
fetchPosts()
}, [])
The above code is running the fetchPosts()
function on page load. Inside the fetchPosts()
function, we send a GET
request to the WordPress API using Axios to retrieve posts and then save to the statewe declared earlier.
Step 4: Creating a Blog Component
Inside of the root directory, create a new folder named components, and inside of it, create two new files: blog.jsx and blog.css.
First, add the following code to blog.jsx:
import axios from "axios";
import React, { useEffect, useState } from "react";
import "./blog.css";
export default function Blog({ post }) {
const [featuredImage, setFeaturedimage] = useState();
const getImage = () => {
axios
.get(post?._links["wp:featuredmedia"][0]?.href)
.then((response) => {
setFeaturedimage(response.data.source_url);
});
};
useEffect(() => {
getImage();
}, []);
return (
<div class="container">
<div class="blog-container">
<p className="blog-date">
{new Date(Date.now()).toLocaleDateString("en-US", {
day: "numeric",
month: "long",
year: "numeric",
})}
</p>
<h2 className="blog-title">{post.title.rendered}</h2>
<p
className="blog-excerpt"
dangerouslySetInnerHTML={{ __html: post.excerpt.rendered }}
/>
<img src={featuredImage} class="mask" />
</div>
</div>
);
}
In the above code, we have created a card component that takes the posts
property that contains the information about the blog post from the WordPress API.
In the getImage()
function, we use Axios to fetch the URL of the featured image and then save that information to state.
Then, we added a useEffect
hook to call the getImage()
function once the component has mounted. We also added the return statement in which we are rendering the post data, title, excerpt, and image.
Next, add the styles below to the blog.css file:
@import url("https://fonts.googleapis.com/css?family=Poppins");
.blog-container {
width: 400px;
height: 322px;
background: white;
border-radius: 10px;
box-shadow: 0px 20px 50px #d9dbdf;
-webkit-transition: all 0.3s ease;
-o-transition: all 0.3s ease;
transition: all 0.3s ease;
}
img {
width: 400px;
height: 210px;
object-fit: cover;
overflow: hidden;
z-index: 999;
border-bottom-left-radius: 10px;
border-bottom-right-radius: 10px;
}
.blog-title {
margin: auto;
text-align: left;
padding-left: 22px;
font-family: "Poppins";
font-size: 22px;
}
.blog-date {
text-align: justify;
padding-left: 22px;
padding-right: 22px;
font-family: "Poppins";
font-size: 12px;
color: #c8c8c8;
line-height: 18px;
padding-top: 10px;
}
.blog-excerpt {
text-align: justify;
padding-left: 22px;
padding-right: 22px;
font-family: "Poppins";
font-size: 12px;
color: #8a8a8a;
line-height: 18px;
margin-bottom: 13px;
}
Then, in the App.jsx file, add the follow code in the return
statement to render the blog component:
<div>
{posts.map((item) => (
<Blog post={item} />
))}
</div>;
Finally, this is what your App.jsx should look like:
import React, { useEffect, useState } from 'react'
import axios from "axios"
import Blog from './components/Blog';
export default function App() {
const [posts, setPosts] = useState([]);
const fetchPosts = () => {
axios
.get("http://my-awesome-website.local/wp-json/wp/v2/posts")
.then((res) => {
setPosts(res.data);
});
}
useEffect(() => {
fetchPosts()
}, [])
return (
<div>
{posts.map((item) => (
<Blog
post={item}
/>
))}
</div>
)
}
Save the file and refresh your browser tab. Now you should be able to see your blog posts rendered on the page.
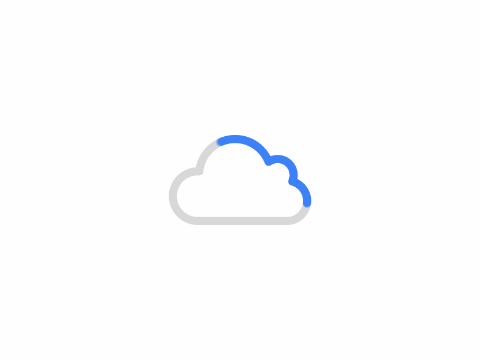
Summary
Headless WordPress offers a great way to create fast-performing websites with a scalable architecture. With the use of React and the WordPress REST API, it is easier than ever to create dynamic and interactive websites with WordPress as the content management system.
Just as important to speed is where you host your WordPress site. Kinsta is uniquely poised to provide a lightning-fast WordPress hosting experience with top-of-the-line Google C2 machines on their Premium Tier Network, as well as a Cloudflare integration to lock your site down against data loss and malicious attacks.
Have you built — or are you planning to build — a headless WordPress website with React? Let us know in the comments section below!
The post How To Create a Headless WordPress Site With React.js appeared first on Kinsta®.
共有 0 条评论